JSON (JavaScript Object
Notation) is well known data encoding format that enables sending/receiving
data from Client to Service and vice versa.
We will see the below
topics in this article with simple steps below,
1. JSON
Serialization - Convert Object to JSON
2. JSON
De-Serialization -
Convert JSON to object.
To do the JSON Serialization/De-Serialization, add the below
references in your project.
ü System.Runtime.Serialization
Step 1: Creation of Data Contract
[DataContract]
public class Person
{
[DataMember]
public string Name { get; set; }
[DataMember]
public int Age { get; set; }
[DataMember]
public int Salary { get; set; }
}
Step 2: JSON Serialization
/// <summary>
/// Serialize Object to JSON.
/// </summary>
private static MemoryStream SerializeToJSON()
{
var person = new Person();
person.Name = "Person name";
person.Age = 10;
person.Salary = 1000;
var ms = new MemoryStream();
DataContractJsonSerializer ds = new DataContractJsonSerializer(person.GetType());
ds.WriteObject(ms, person);
ms.Position = 0;
StreamReader sr = new StreamReader(ms);
Console.Write("*** --- Object Serilization To JSON
Format--- ***\n");
Console.WriteLine(sr.ReadToEnd());
Console.Write("\n");
return ms;
}
Step 3: JSON De-Serialization
/// <summary>
/// DeSerialize JSON To Object
/// </summary>
/// <param
name="ms"></param>
private static void DeSerializeToJSON(MemoryStream ms1)
{
Console.WriteLine("*** --- JSON Deserilization To Object --- ***\n");
var ms = new MemoryStream(ms1.ToArray());
DataContractJsonSerializer ds1 = new DataContractJsonSerializer(typeof(Person));
XmlDictionaryReader dr = JsonReaderWriterFactory.CreateJsonReader(ms, new XmlDictionaryReaderQuotas());
var person = (Person)ds1.ReadObject(dr);
Console.WriteLine("Name: " + person.Name);
Console.WriteLine("Age: " + person.Age);
Console.WriteLine("Salary: " + person.Salary);
}
Testing It: 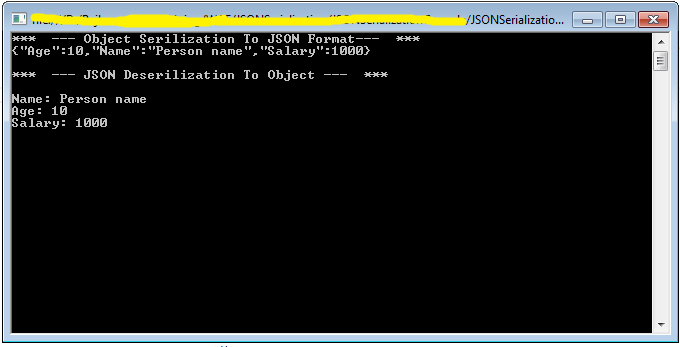
|