This article explains about Data “Serialization and De-Serialization of an Object” in WCF with very easy steps.
Step 1: Create a Model Class
public class Student
{
public string Name { get; set; }
public double Salary { get; set; }
public string Address { get; set; }
}
Step 2: Use “DataContractSerializer” to serialize object into XML.
private static void Serialize(string filename)
{
var student = new Student();
student.Name = "TestName";
student.Salary = 700;
student.Address = "TestAddress";
using (FileStream ioStream = new FileStream(filename, FileMode.Create))
{
DataContractSerializer serializer = new DataContractSerializer(typeof(Student));
serializer.WriteObject(ioStream, student);
}
}
DataContractSerializer will write the object in the XML formation in the given path. 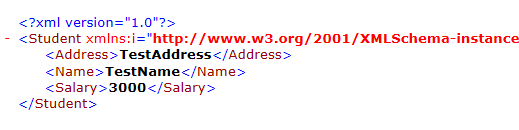
Step 3: Use “DataContractSerializer” & “XmlDictionaryReader” to de-serialize xml to Object.
private static void DeSerialize(string fileName)
{
using (FileStream ioStream = new FileStream(fileName, FileMode.Open))
{
DataContractSerializer serializer = new DataContractSerializer(typeof(Student));
XmlDictionaryReader xmlDictonaryReader = XmlDictionaryReader.CreateTextReader(ioStream, new XmlDictionaryReaderQuotas());
var student = (Student)serializer.ReadObject(xmlDictonaryReader);
xmlDictonaryReader.Close();
Console.WriteLine("Name : " + student.Name);
Console.WriteLine("Salary : " + student.Salary);
Console.WriteLine("Address :" + student.Address);
}
} OutPut 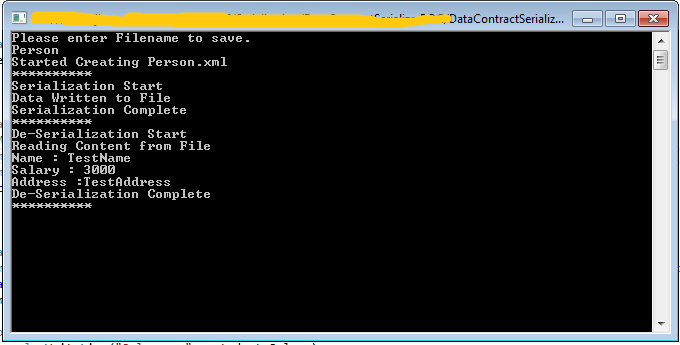
|