The
serialization and its uses are as follows.
Serialization is the process of converting an object into
stream of bytes in order to store the object in a file, Memory, Database and in
transferring the object over the network. The reverse process is called as
De-Serialization. Please find the below Fig. 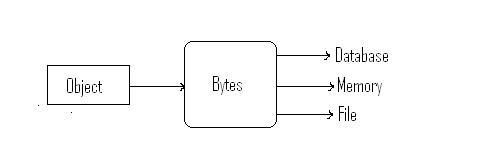
In order to achieve this
serialization, the class should be encapsulated with [Serializable] attribute. Without this
attribute, “Serialization” is not possible. If the base class is derived, then
the both class should be encapsulate with [Serializable]
attribute to achieve the serialization.
Given below the serialization example by using two classes.
namespace
SerializationExample
{
[Serializable]
public class EmployeeSkill
{
public string Skill { get; set; }
}
[Serializable]
public class Employee : EmployeeSkill
{
public string Name { get; set; }
public int Experience { get;
set; }
}
}
By using the above two
classes I am going to show how to,
1.
Convert an object into bytes and vice versa.
2.
Serialize an object to save and retrieve the
object from the text file.
3.
Serialize an object to save and retrieve the
object from the XML file.
1.
Convert
an object into bytes and vice versa.
namespace
SerializationExample
{
class Program
{
static void Main(string[]
args)
{
var
objSerializationObject = new Employee()
{
Name = "Employee",
Experience = 3,
Skill = ".Net"
};
var
objConvertedToBytes = ConvertObjectToBytes(objSerializationObject);
var
objEmployee = (Employee)ConvertBytesToObject(objConvertedToBytes);
Console.WriteLine(objEmployee.Name);
Console.WriteLine(objEmployee.Experience);
Console.WriteLine(objEmployee.Skill);
Console.ReadLine(); }
#region Converting Object
into Bytes
/// <summary>
/// To convert object into Bytes.
/// </summary>
/// <param
name="objEmployee">Employee
Object</param>
/// <returns>Array Of Bytes</returns>
public static byte[]
ConvertObjectToBytes(Employee objEmployee)
{
if
(objEmployee == null) return
null;
MemoryStream
objMemoryStream = new MemoryStream();
BinaryFormatter
objBinaryFormatter = new BinaryFormatter();
objBinaryFormatter.Serialize(objMemoryStream, objEmployee);
return
objMemoryStream.ToArray();
}
#endregion
#region Converting Bytes
into Original Object
/// <summary>
/// To convert bytes into Object.
/// </summary>
/// <param
name="objArrayBytes">Array Of
Bytes</param>
/// <returns>Original Object</returns>
public static Object
ConvertBytesToObject(byte[] objArrayBytes)
{
MemoryStream
objMemoryStream = new MemoryStream();
BinaryFormatter
objBinaryFormatter = new BinaryFormatter();
objMemoryStream.Write(objArrayBytes, 0, objArrayBytes.Length);
objMemoryStream.Seek(0, SeekOrigin.Begin);
Object
obj = (Object)objBinaryFormatter.Deserialize(objMemoryStream);
return
obj;
}
#endregion
}
}
2.
Serialize to save and retrieve an object from the text file.
namespace
SerializationExample
{
class Program
{
static void Main(string[] args)
{
var objSerializationObject = new Employee()
{
Name = "Employee",
Experience = 3,
Skill = ".Net"
};
#region
Saving the object stream in the text file
IFormatter objFormatter = new BinaryFormatter();
Stream objStream = new FileStream("MyFile.txt", FileMode.Create,
FileAccess.Write, FileShare.None);
objFormatter.Serialize(objStream, objSerializationObject);
objStream.Close();
#endregion
#region
Read and Convert the Stream of bytes into original Object
IFormatter objReadFormatter = new BinaryFormatter();
Stream objReadStream = new FileStream("MyFile.txt", FileMode.Open,
FileAccess.Read, FileShare.None);
Employee objDeserialize = (Employee)objReadFormatter.Deserialize(objReadStream);
objReadStream.Close();
#endregion
Console.WriteLine(objDeserialize.Name);
Console.WriteLine(objDeserialize.Experience);
Console.WriteLine(objDeserialize.Skill);
Console.ReadLine();
}
}
}
3.
Serialize
to save and retrieve an object from the XML file.
namespace
SerializationExample
{
class Program
{
static void Main(string[]
args)
{
var objSerializationObject = new Employee()
{
Name = "Employee",
Experience = 3,
Skill = ".Net"
};
#region
Saving the object stream in the XML file
XmlSerializer objXMLSerializer = new XmlSerializer(typeof(Employee));
StreamWriter objWriter = new StreamWriter("MyFile.XML");
objXMLSerializer.Serialize(objWriter, objSerializationObject);
objWriter.Close();
#endregion
#region
Read the XML and Convert it into original Object
StreamReader objReader = new StreamReader("MyFile.XML");
Employee objDeserialize = (Employee)objXMLSerializer.Deserialize(objReader);
objReader.Close();
#endregion
Console.WriteLine(objDeserialize.Name);
Console.WriteLine(objDeserialize.Experience);
Console.WriteLine(objDeserialize.Skill);
Console.ReadLine();
}
}
}
|